Three small changes that will improve your style in Python
I often come across code that works but it takes more effort to read because the author doesn’t know yet about parts of the language or library. Because the code works, it’s hard to improve on the style unless someone presents another way of writing the same thing.
So here comes my edition #1 of Python tips.
1. if True
Consider this snippet:
if (result == True):
do_something()
else:
do_another_thing()
This is strictly equivalent to:
if result:
do_something()
else:
do_another_thing()
Comparing something
to True
only converts something
to boolean but if
does that
anyway. Also, parentheses around the whole expression hurt readability a bit.
In a similar way, if result == False
reads more natural as if not result
.
A variant of this is:
if x < 3:
v = True
else:
v = False
Which can be simplified to
v = x < 3
2. Comment on intent, not on what’s in the code
# Create lookup dict
name_lookup_dict = <... some complicated expression, maybe with Pandas ...>
The comment here doesn’t add much value. The author correctly used the variable name for explaining what it is. What could a comment add here? Because Python is dynamically typed, it’s useful to capture what’s inside of the variables if their construction is not obvious.
This comment, together with the variable name tells us what do we want to have in the variable.
# email => name + " " + surname
name_lookup_dict = <...>
3. Starts with, ends with
This code works:
in_documents = os.path.abspath('.')[-9:] == 'Documents'
But this code speaks more clearly about the intent and you don’t have to calculate the length of your fixed string:
in_documents = os.path.abspath('.').endswith('Documents')
The string method name endswith
makes the intent easier to read.
One potential drawback here is that you get a false positive if the user is in directory
e. g. OldDocuments
. There’s a more modern library in Python for working with paths
specifically:
from pathlib import Path
in_documents = (Path.cwd().name == 'Documents')
Unfortunately, the last snippet relies on the reader knowing what
Path.name
means (the last component of the path), so it’s a bit harder to read.
Conclusion
Please don’t reject reviews (or pull request) if you see code I recommend here to change. It’s usually a waste of time to argue about these smaller style differences.
On the other hand, point out ways how people can improve their code writing style. If you can do it without being a smart-ass, everyone will benefit.
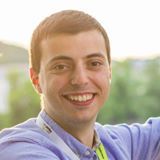
filip at sedlakovi.org - @koraalkrabba
Filip helps companies with a lean infrastructure to test their prototypes but also with scaling issues like high availability, cost optimization and performance tuning. He co-founded NeuronSW, an AI startup.
If you agree with the post, disagree, or just want to say hi, drop me a line: filip at sedlakovi.org